I attended Clojure/conj this past weekend and I wanted to recollect my
notes and thoughts on my experience to share with others who weren’t able to
make it.
The Venue
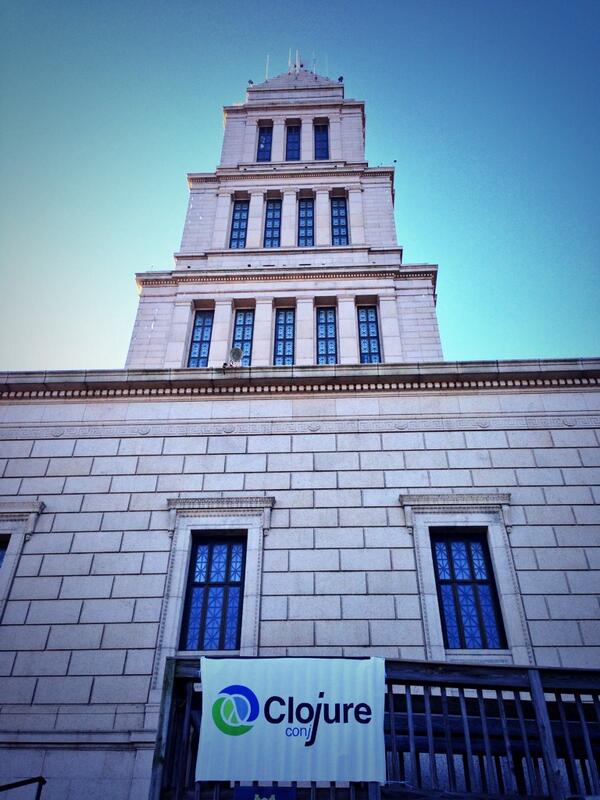
The event was held at the George Washington Masonic Memorial, a landmark
structure in Alexandria, Virginia.
Being a VA native, I have seen this building several times while visiting the
area, but never been inside. Even if I had, I don’t think I’d imagine that it
would be a good spot to host a programming conference, but it was.
There was a single stage with theater seating — the acoustics were great and
it seemed to be exactly the right size. Not being able to bring food & drinks
(i.e., caffeine) into the theater might have been the only inconvenience, but
totally understandable given the significance of the building.
The Talks
Day 1
Stu Halloway – data.fresssian
Stu kicked off the conference by introducing a new data encoding library,
data.fressian (pronounced ‘data freshen’), that’s being used
in Datomic to efficiently transport data across the
wire.
It has similar properties to edn, like
being language agnostic, self-describing, and extensible, but in a binary
format. The library’s designers prioritized effectiveness before efficiency to
avoid common idiosyncrasies found in existing (JVM) serialization libraries.
Checkout the clojure implementation and the
format’s wiki for more information.
Update: video
Mike Anderson – Enter the Matrix
Mike presented the core.matrix API for matrix and vector
operations. At its core, the library is a set of protocols that abstract both
fundamental (required) and composite (optional) matrix operations. This allows
any of the existing matrix libraries for the JVM to be leveraged.
By providing a standard API to build applications and leaving the underlying
matrix engine pluggable, you can optimize for your particular use case.
Since this is all protocol-based, you could conceivably mix & match them.
core.matrix currently ships with protocol extensions for vectorz-clj, Clatrix
and NDArray.
Update: video
Aria Haghighi – Prismatic’s Schema
I was looking forward to this talk about Prismatic’s schema library,
as I had seen their introductory blog post and started playing
with it on my flight to the conference. Schema, as its name suggests, is a way
to describe and validate the shape of your data.
Aria made the point that while it’s certainly possible to infer the
shape of data from the source code or convey with a docstrings, it’s time
consuming and imprecise even in the most trivial of situations. Schemas provide
a clear and concise documentation that is non-intrusive like static type systems
can be. He reported noticeable productivity gains at Prismatic from using
schema and simple code organizational patterns.
Aside from documentation and validation, he mentioned that schemas can be used
for other interesting things, like code generation. Prismatic has been using
schema to generate ObjectiveC and Java code for their mobile iOS and Android
applications, which they plan to open source in the future.
Update: video
Chris Houser & Jonathan Claggett – Illuminated Macros
Clojure has powerful macro facilities, but they can be hard to debug when something
goes wrong. There aren’t many ways for a macro’s author to provide fine-grained
error messages or suggested fixes. And aside from macroexpand
, macros
can be opaque to inspect and understand.
Chouser presented properties of macros and ideas that we can
learn from (good parts of) Racket’s macros. And Jonathan demoed
seqex, a library for “s-expression regexes.”
He gave examples by describing the syntax for the let
macro that provides helpful
feedback to users and even generates a colorized, complete syntax specification.
It would be really cool to see some of these patterns become popular in Clojure.
Update: video
Nada Amin & William Byrd – From Greek to Clojure!
A talk that was equally entertaining as it was thought-provoking;
Nada and Will gave tips on how to approach technical
research papers with some live coding using core.logic.
Update: video
Rich Hickey – Harmonikit
Rich took a break from his normal keynote this year to present a
“fun” project that he’s been working on. Harmonikit is a musical synthesis
system built with core.async, overtone,
Supercollider, Lemur and
a keyboard controller that I didn’t catch the name of.
As always, Rich did an exemplary job of dissecting & describing the problem
domain, choosing the distinct problems he wants to address and modeling a system
to do it. This system focused on realistically synthesizing acoustics of harmonic
(e.g., stringed, wind) instruments.
I won’t do this project & presentation justice if I continue attempting to
describe it, so I’ll spare you (and myself) and let you check out the recording
when it is released.
Update: video
Day 2
Carin Meier – Learning to Talk to Machines with Speech Acts
Carin is a great presenter and story teller. She told a story
illustrating how computer science can learn from philosophy and vice versa. She
did an awesome demo of a flying drone using babar and
clj-drone, and of course, now I want a drone.
Update: video
Timothy Baldridge – core.async
Tim skipped talking about the rationale of
core.async, which is well documented elsewhere,
and jumped right into the usage and mechanics of the library that has recently
taken the Clojure & ClojureScript communities by storm. I’ve been using this
library in a side-project, yet learned several new things like the
size of the threadpool.
Update: video
Michael O’Keefe – Predicting Vehicle Usage with Clojure
Michael is a mechanical engineer by trade who has written
several vehicle efficiency simulators
in the past, with
his most recent being in Clojure. He had helpful graphics of the simulation model
and totally awesome original illustrations. It seemed that Clojure’s
immutable values and ease of building dataflows via functional composition were
significant advantages of this type of system.
Interestingly, he was also the only presenter to use LightTable editor during a
demo (AFAIK), which was very easy to follow (one presenter used vim, and the
rest used emacs)
Update: video
Tim Ewald – Clojure: Programming with Hand Tools
This was one of the most memorable talks I’ve been to. This wasn’t a Clojure or
even programming specific talk. Instead, Tim shed some light on the craft of
woodworking, particularly with hand-tools rather than than power-tools. Here
are a few of the observations he made that I can remember:
- The less you do, the less you know.
Automating a complex process isn’t a bad thing in and of itself, but it can
build reliance on machines to do a job; humans begin to lose the skills and
appreciation for the task at hand.
- Your tools change the way you perceive the world.
This is related to the “if all you have is a hammer, everything begins to
look like a nail” lesson. Confirmation bias leads to bad decisions that
don’t appear bad on the surface.
- Simpler tools give you a broader perspective.
You are closer to your trade when your tools allow you (humans) to create
unique items, leaving automation to handle the tasks that never change.
Regardless of how archaic hand-tools may seem, it can be impossible to
match the level of precision that they provide.
Looking forward to watching the recording of this talk.
Update: video
Day 3
Thomas Kristensen – Propagators in Clojure
Thomas presented propaganda, a library for
declarative logic programming with propagators. It provides similar
functionality as core.logic, but benefits from a more transparent
processing model and has efficiency benefits for numerical problems.
Update: video
Fogus – Zeder: Production Rule Systems in Clojure
Zeder is a production rule system
(think OSP5), that Fogus has been
building. The syntax was very similar to Datomic’s and looks very powerful.
He doesn’t appear to have released the source for it yet, but I did find a
gist with an example.
Update: video
Russ Olsen – To the Moon!
Russ gave a vivid recollection of America’s race to
the moon in the 60’s and the little and big lessons we can learn from it today.
The talk’s theme was centered around one of JFK’s quotes, “We choose to go to
the moon, not because it’s easy, but because it’s hard.”
As a Millennial, I’ve heard and seen this story in popular culture countless
times, but he covered some amazing context & details that most leave out.
Russ is a great speaker and the story was inspirational.
Definitely watch this talk when the video is released.
Update: video
Last but Not Least
There were a handful of talks not mentioned here, including
some lightning talks. One of which was from Alan Dipert on
gherkin, aka bash killer. I also got a kick out of talks by
Steve Miner and Amit Rathore.
Update: video
Final Words
Huge thanks to conference organizers, Alex Miller and
Lynn Grogan, for your hard work and leadership. I am immensely
grateful.
And, of course, thanks to all of the speakers, as well as the sponsors, for
making it happen. For me, this has been a real testament to the authenticity of
the Clojure community.
Lastly, I wanted to give a big thank you to my employer,
Huddler, for sponsoring me to attend this conference
(and letting me work in Clojure).
Until next year!